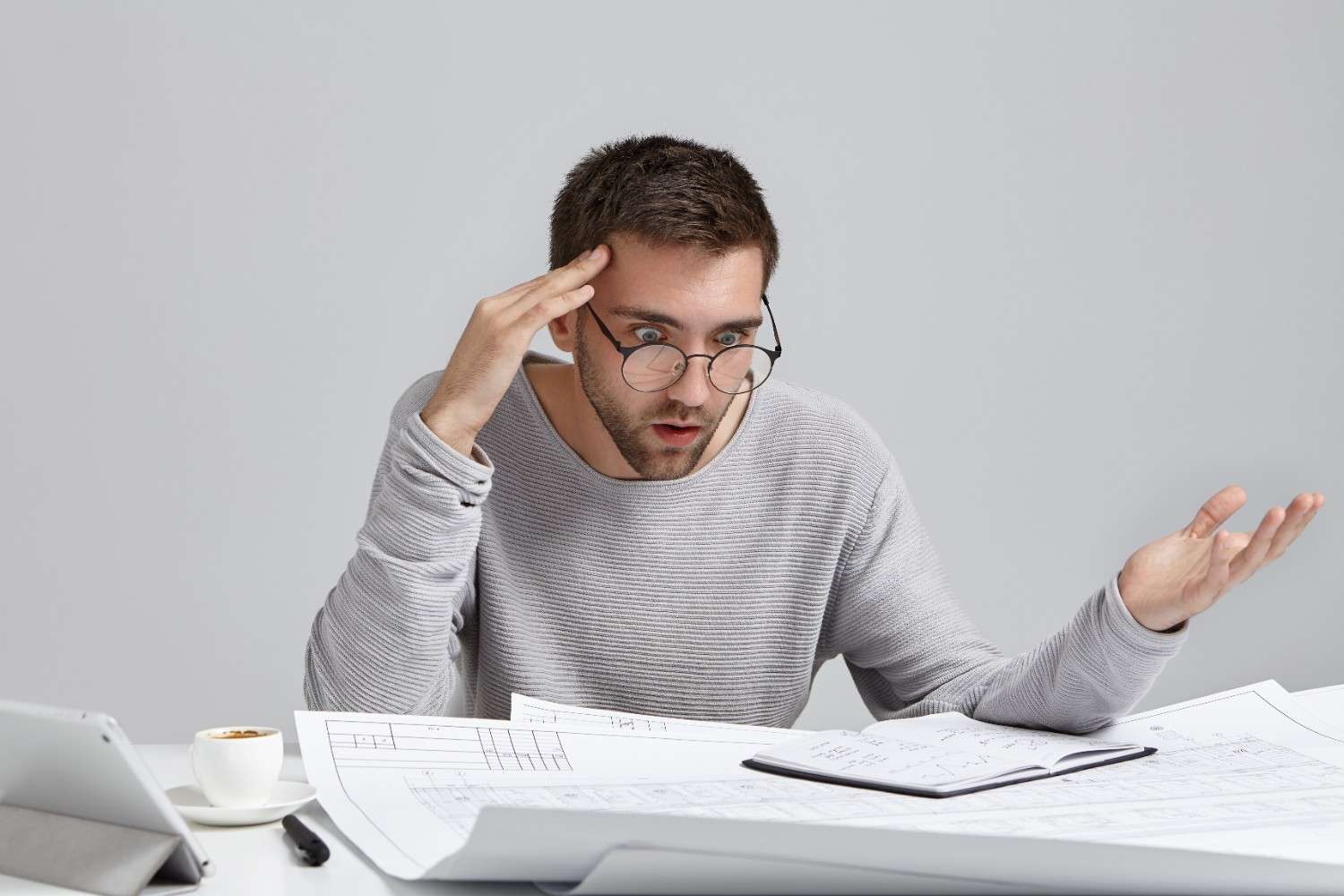
ReactJS is one of the most flexible and powerful JavaScript libraries for rendering user interfaces. It is popularly used for creating applications on single pages. Even a seasoned ReactJS developer faces a few errors in his career with ReactJS, which can be annoying, but they are surprisingly simple because mere understanding of the error causes makes it possible to solve it quite easily.
Thus the article discussed some of the common ReactJS errors with their causes and solutions. This article is useful to new and experienced developers for troubleshooting and writing cleaner and more efficient code with React.
Understanding the Errors in ReactJS
ReactJS usually classifies its errors into two types:
– Compilation Errors- The application does not develop code because of syntax faults and missing dependency issues. It mostly occurs in incorrect configuration.
– Runtime Errors- As the name suggests, these happen when the application is up and running. These still consist of undefined variables or not having the right state assigned and not involving proper component usage.
Addressing these errors effectively requires an understanding of both the React framework and JavaScript fundamentals. Let’s delve into some of the most common errors and their solutions.
Error “Module Not Found”
Cause
This error occurs when a required module or file cannot be located. It’s often due to:
Incorrect import paths.
Missing dependencies in your project.
A typo in the filename or path.
Solution
Double-check your import statements to ensure the file paths are correct.
Ensure the file or module exists in the specified location.
Run npm install or yarn install to ensure all dependencies are installed.
If the issue persists, clear the node_modules folder and reinstall dependencies:
rm -rf node_modules
npm install
2. Error: “Cannot Read Property of Undefined”
Cause
This error occurs when your code tries to access a property of an undefined object. Common scenarios include:
A variable hasn’t been initialized.
An asynchronous operation hasn’t completed yet.
Solution
Use optional chaining (?.) to safely access object properties:
console.log(user?.profile?.name);
Add null checks to ensure the object exists before accessing its properties:
if (user && user.profile) {
console.log(user.profile.name);
}
Debug the code to find where the undefined value originates.
Error: “Invalid DOM Property”
Reason
React keeps a very strict standard about the HTML attributes. For example, class in HTML is className in JSX.
Solution
All HTML attributes must be written correctly in JSX. Check the correct syntax in the React documentation.
For example, incorrect attributes may be replaced as follows:
// Incorrect
// Correct
4. Error: “React Hook UseEffect Has a Missing Dependency”
Reason
The warning occurs whenever the useEffect hook’s dependencies array is incomplete and this is used by React to determine the re-running of effects.
Solution
Consider including all variables that are used inside the useEffect hook in the dependency array:
useEffect(() => {
fetchData();
}, [fetchData]);
You can also use the eslint-plugin-react-hooks package to identify missing dependencies.
5. Error: “Rendered More Hooks Than During the Previous Render”
Reason
This error occurs when the count of hooks invoked in renders keeps varying.Common reasons include:
Placing hooks inside conditional statements or loops.
Solution
Ensure all hooks are called unconditionally, at the top level of your component:
if (condition) {
const [state, setState] = useState(0); // Incorrect
}
const [state, setState] = useState(0); // Correct
if (condition) {
// Do something
}
6. Error: “React.Children Only Expected to Receive a Single Child”
Cause
This happens when a component expecting a single child receives multiple children.
Solution
Wrap multiple children in a React Fragment or a
:return (
.Error: “Maximum Update Depth Exceeded”
Cause
This error occurs when a component enters an infinite rendering loop, usually due to a state update triggering another state update.
Solution
Check for unintended state updates in your component logic.
Use functional state updates when the new state depends on the previous state:
setCount((prevCount) => prevCount + 1);
8. Error: “A Component is Changing an Uncontrolled Input to Be Controlled”
Cause
This error occurs when an input element switches between controlled and uncontrolled modes.
Solution
Ensure the value of the input is always defined:
setValue(e.target.value)} />
9. Error: “TypeError: Cannot Add Property, Object is Not Extensible”
Cause
This happens when you try to add a property to a frozen object.
Solution
Avoid directly modifying objects. Use immutability helpers or the spread operator:
const updatedObj = { …obj, newProp: ‘value’ };
10. Error: “Expected an Assignment or Function Call and Instead Saw an Expression”
Cause
This error occurs when you write an expression that doesn’t produce a side effect, such as using a statement like x + y; instead of an assignment or function call.
Solution
Ensure all expressions in your code have a purpose:
const sum = x + y; // Correct
Tips for Avoiding Common React Errors
1. Keep Dependencies Updated
Outdated packages often introduce compatibility issues. Regularly update your dependencies using:
npm update
2. Leverage Linting Tools
Linting tools like ESLint can catch potential errors in your code before runtime.
3. Use React DevTools
React DevTools is invaluable for debugging component trees and state issues. Install it as a browser extension for Chrome or Firefox.
4. Follow Best Practices
Adopting best practices like clean code architecture, modular components, and proper state management can significantly reduce errors.
Final Thoughts
ReactJS errors can seem daunting at first, but understanding their causes makes resolving them much easier. Following best practices, keeping your code clean, and leveraging debugging tools like React DevTools can significantly reduce the frequency of these errors.
By proactively addressing common issues and learning from these errors, you’ll become more proficient in React development. Bookmark this guide for quick reference, and happy coding!