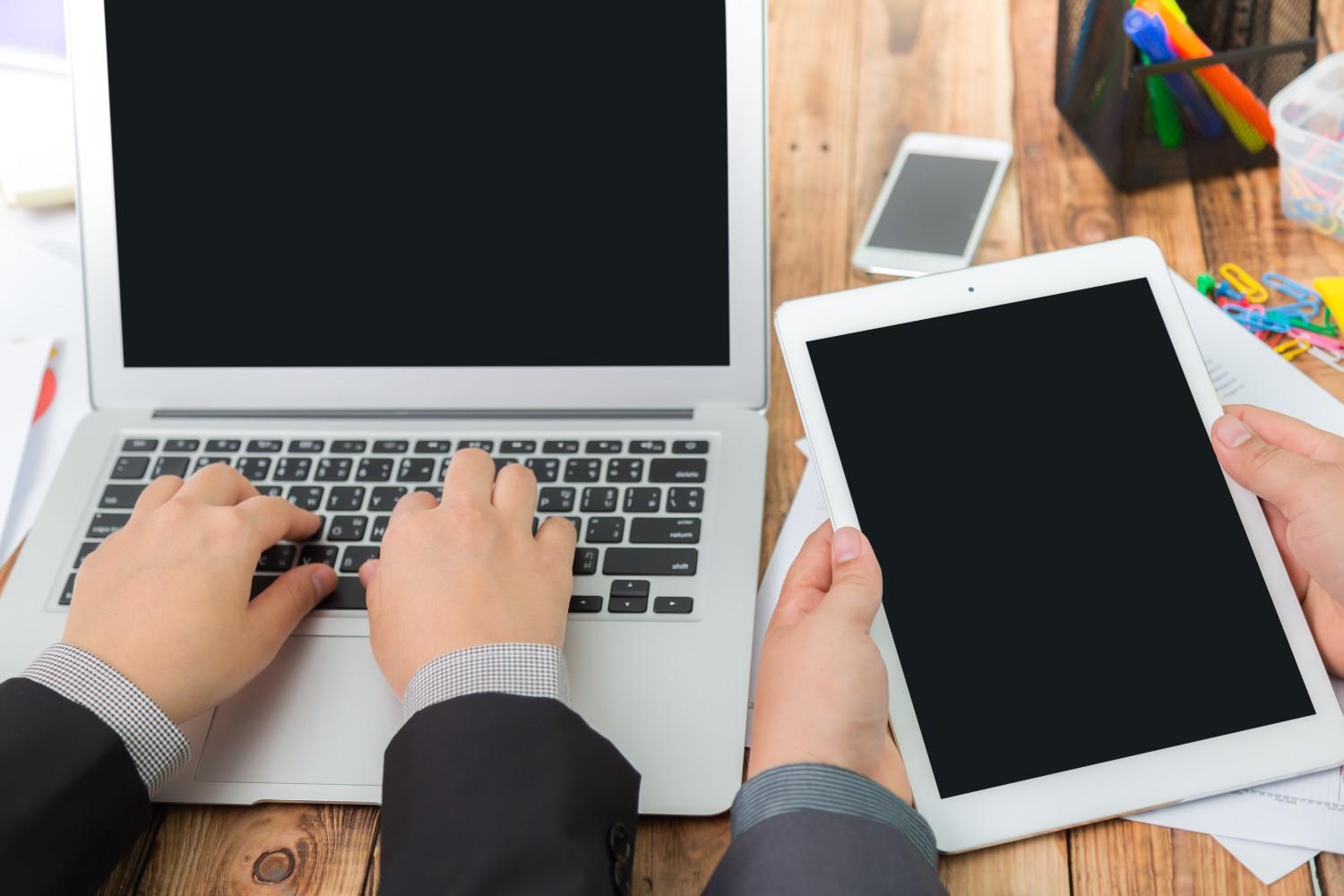
In today’s digital landscape, having a responsive website is essential for delivering an optimal user experience across devices. ReactJS, a popular JavaScript library for building user interfaces, provides the tools needed to create responsive and dynamic websites efficiently. This guide will walk you through the process of building a responsive website using ReactJS, covering essential techniques, best practices, and tools to ensure your project is SEO-friendly and adheres to Google AdSense policies.
What is a Responsive Website?
A responsive website adapts its layout and design based on the screen size, resolution, and orientation of the user’s device. This ensures a seamless browsing experience, whether users access your site on a desktop, tablet, or smartphone. Key elements of a responsive design include:
Fluid grids and layouts
Scalable images and media
Media queries for CSS
Optimized performance for all devices
Why Choose ReactJS for Responsive Websites?
ReactJS offers several advantages for creating responsive websites:
Component-Based Architecture: Break your UI into reusable components, making it easier to manage and scale.
React Hooks: Simplify state and lifecycle management, enabling dynamic behavior.
Integration with CSS-in-JS Libraries: Tools like Styled Components and Emotion allow seamless styling.
Third-Party Libraries: Access to libraries like React Router for navigation and Material-UI for responsive design components.
Fast Rendering: React’s virtual DOM ensures efficient updates, improving performance on all devices.
Steps to Create a Responsive Website Using ReactJS
Set Up Your ReactJS Project
First, create a new ReactJS project using Create React App or a similar tool.
npx create-react-app responsive-website
cd responsive-website
npm start
This command sets up a boilerplate React application with essential configurations.
Step 2: Design the Layout
Use a mobile-first approach to design your layout. Break your UI into components such as Header, Footer, Sidebar, and ContentArea.
Example file structure:
src/
|– components/
|– Header.js
|– Footer.js
|– Sidebar.js
|– ContentArea.js
Make Responsive CSS Using Media Queries
Create diverse styling within media queries based on the dimensions of the devices. So create a styles.css file and add breakpoints using media queries.
body {
margin: 0;
font-family: Arial, sans-serif;
}
.container {
display: flex;
flex-wrap: wrap;
}
/* Small screens */
@media (max-width: 768px) {
.container {
flex-direction: column;
}
}
/* Larger screens */
@media (min-width: 769px) {
.container {
flex-direction: row;
}
}
Use CSS Frameworks or Libraries
This may considerably speed up the whole development process. It normally provides responsive design frameworks like Material-UI or Bootstrap.
Using Material-UI:
Install the library:
npm install @mui/material @emotion/react @emotion/styled
Create a responsive grid:
import { Grid, Box } from ‘@mui/material’;
function ResponsiveLayout() {
return (
Box 1
Box 2
Box 3
);
}
Use React Hooks for Dynamic Behavior
React Hooks like useState and useEffect enable dynamic adjustments. So you can read the dimensions of the viewport to enable dynamic-layout switching.
import { useState, useEffect } from ‘react’;
function ResponsiveComponent() {
const [isMobile, setIsMobile] = useState(
window.innerWidth < 768 ); useEffect(() => {
const handleResize = () => {
setIsMobile(window.innerWidth < 768); }; window.addEventListener(‘resize’, handleResize); return () => window.removeEventListener(‘resize’, handleResize);
}, []);
return (
:
Desktop Layout
}
);
}
Optimize all images and media files.
Implementation of srcset and lazy loading to optimize those responsive images, which can also be integrated with libraries such as react-lazy-load for the purpose of performance optimization.
Create import LazyLoad from react-lazyload.
function ResponsiveImages(){
return(
);
}
Step 7: Test for Responsiveness
Screen sizes in browser developer tools- For eg. Chrome DevTools or Lighthouse with which performance and responsiveness can be analyzed.
Advantage of SEO
Meta Tags: Specify such meta tags as viewport for mobile compatibility. example:
Faster Load Time: Optimize JavaScript, CSS, and media files for faster loading speeds.
Accessible All Contents: Ensure access to all text, image, and even interactive objects.
Google AdSense Integration
Ad Placement: Integrate ads while not blocking navigation or content.
Design Mobile-friendly: Check that ads render well to different screen outputs.
Content Quality: Users should not be bombarded with ads as it spoils their experience.
Conclusion
This is one of the quickest and most effective methods to make a site responsive using ReactJS: support a great user experience across devices. React’s component-based architecture combined with CSS frameworks and coding best practices would allow a developer to build modern-day responsive websites that pass the SEO and AdSense tests. To build everything, just follow the above steps to create a highly professional, high-performance site according to today’s web standards.