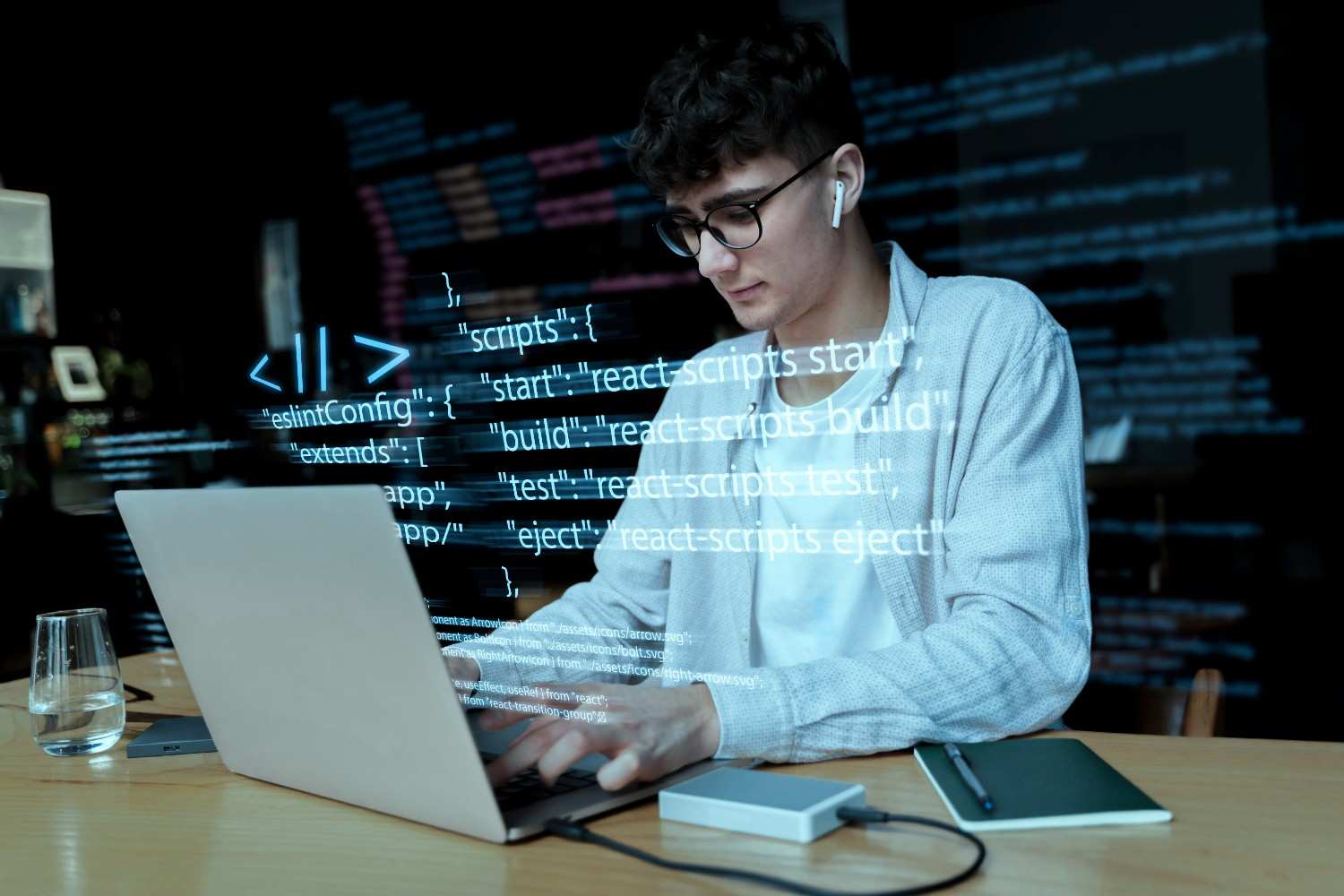
Built and maintained by Facebook, ReactJS is currently among the most popular JavaScript libraries for the construction of dynamic user interfaces. The component-based architecture and the declarative nature of ReactJS make it the most preferred even by entry-level developers as well as very advanced ones. For the new learner, there is nothing more exciting than creating the first application using React; it has the beauty of coming to the new web world’s horizon. This will take you through the process of creating your very own first app while making sure it is fully functional; in addition, it will take due care of Google AdSense policies as well as SEO best practices.
What indeed is ReactJS?
ReactJS is a JavaScript library, designed with special focus on developing single-page applications. Unlike traditional web development frameworks, React allows developers to create things called encapsulated components, which take care of their own state. These components can then be brought together to make fairly complicated user interfaces without much pain.
Key features of ReactJS include:
Component-Based Architecture: Write reusable components that simplify UI design.
Virtual DOM: React’s Virtual DOM optimizes performance by minimizing direct updates to the real DOM.
Declarative Syntax: React makes it easy to design interactive and dynamic UIs with minimal code.
Rich Ecosystem: Enhanced functionalities can be derived from adjacent supportive tools like Redux, React Router, etc.
Basic Bill of Materials Required in Building a React Application
In prerequisites for building a React app, some of them include:
Basic JavaScript Understanding: And you have to know the ES6 syntactic structure of JavaScript.
Installed Node.js and Npm: They will manage the dependencies for your project and run in performing the functions of your React application.
Common Code Editors: VS Code is a popular code editor to use for React development.
Stepwise Guide to Building Your First React App
Setting Up the Development Environment
To begin, you need to set up your development environment:
Install Node.js from Node.js official website.
Verify the installation:
node -v
npm -v
Install the React app scaffolding tool, Create React App, using npm:
npx create-react-app my-first-app
This command will create a directory named my-first-app with all necessary files and dependencies.
Understanding the File Structure
Once the installation is complete, navigate to your project folder:
cd my-first-app
You’ll see a folder structure like this:
public/: Contains static files like index.html.
src/: Holds the main codebase, including components and styles.
node_modules/: Contains all project dependencies.
3. Running Your React App
Start the development server with:
npm start
This will launch your app in the browser at http://localhost:3000/. You’ll see the default React welcome page.
Creating Your First Component
In React, the UI is built using components. Let’s create a simple component:
Navigate to the src folder and create a new file named Welcome.js:
import React from ‘react’;
function Welcome() {
return (
Welcome to My First React App!
);
}
export default Welcome;
Import this component into App.js:
import React from ‘react’;
import Welcome from ‘./Welcome’;
function App() {
return (
);
}
export default App;
Save the files and view the changes in your browser.
5. Adding Interactivity with State
React components can hold and manage data using state. Let’s add a button that toggles a message:
Modify Welcome.js to include state:
import React, { useState } from ‘react’;
function Welcome() {
const [showMessage, setShowMessage] = useState(false);
return (
Welcome to My First React App!
{showMessage &&
Hello, React World!
}
);
}
export default Welcome;
Save the file and test the functionality.
6. Styling Your App
You can style your app using CSS. Create a new CSS file Welcome.css:
h1 {
color: #4caf50;
}
button {
background-color: #4caf50;
color: white;
border: none;
padding: 10px 20px;
cursor: pointer;
margin-top: 10px;
}
button:hover {
background-color: #45a049;
}
Import this CSS file into Welcome.js:
import ‘./Welcome.css’;
SEO Best Practices for Your React App
Use Descriptive Meta Tags: Ensure public/index.html contains meta tags for title, description, and keywords.
Optimize Images: Use optimized image formats and tools like lazy loading.
Ensure Mobile Responsiveness: Test your app on various screen sizes.
Leverage Server-Side Rendering (SSR): Tools like Next.js can improve SEO by rendering pages on the server.
Adherence to Google AdSense Policies
To monetize your React app with Google AdSense, follow these tips:
Create Quality Content: Ensure your app provides value and does not solely focus on displaying ads.
Avoid Excessive Ads: Maintain a balance between content and ads.
Mobile Optimization: Ensure ads are responsive and non-intrusive on mobile devices.
Comply with Content Policies: Avoid prohibited content such as copyrighted material or misleading claims.
Conclusion
Building your first React app is a rewarding experience that opens the door to endless possibilities in web development. By following this guide, you’ll not only create a functional app but also ensure it adheres to best practices for SEO and Google AdSense. As you gain confidence, you can explore advanced features like API integration, state management with Redux, and more. Happy coding!